Selenium with docker – Part 1
Automation testing has become integral part of any software development cycle. And when it comes to web application automation, the Selenium Web driver is the first thing that comes to mind. Selenium is used to test web applications on different browsers and different platforms. While tests can be run on your local machine, but our requirement is always to run our tests on different OS and different browser versions. To run the tests on different versions of OS or browser we need to maintain different machines with different configurations. This is where Docker comes in handy.
What is Docker and why should we use it for the selenium test?
Docker is an open platform for developing, shipping, and running applications. Docker enables you to separate your applications from your infrastructure so you can deliver software quickly.
When we use Selenium docker, we don’t need to manage different browser versions on different OS. Docker manages its own, using images. Below are the key benefits of using docker with Selenium.
- Highly scalable: When you want to run tests in parallel, you can create as many containers as required for your tests with just one command.
- Easy to Start: Starting a docker container is very easy.
- No need to manage the selenium jar on different machines because it is managed by selenium Docker itself.
- No need to download the browser driver and setting up the path. Docker manages the browser driver itself.
Selenium test can be run on standalone container or grid of multiple containers. In this article, we are going to see how to run selenium tests on standalone container.
Getting Started
Install docker
If you have not installed Docker yet on your machine, please install it. Refer to this documentation.
Setup Selenium test to run on container
We’ll use the below code to run on the container. This code will open https://www.google.co.in on google chrome and will verify the page title. Because we are not running our test on the host machine but on the container, we need to connect to a container IP address using Remote WebDriver. For now, I am taking http://localhost:4444/wd/hub as the remote URL because, in the next steps, I am going to launch the docker container on port 4444 only.
WebDriver driver;
ChromeOptions chromeOptions = new ChromeOptions();
driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), chromeOptions);
driver.get("https://www.google.co.in");
Assert.assertEquals(driver.getTitle(), "Google");
Setup docker container for selenium test
- Pull the docker image for chrome browser using the below command. This will download the latest image for standalone chrome.
docker pull selenium/standalone-chrome
Once the image pull is complete, you will see logs like the below screenshot in your terminal/cmd
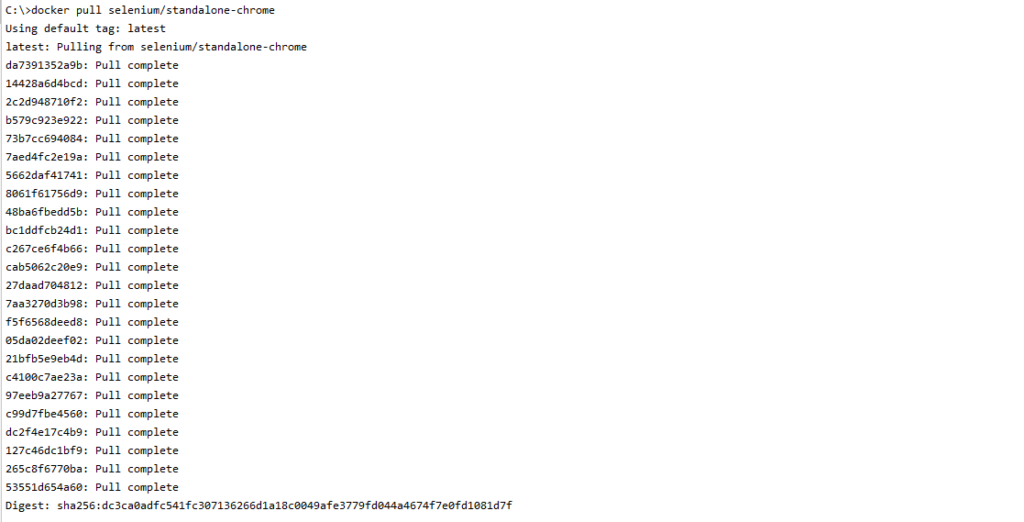
- Once the image is downloaded successfully, verify the same using the below command. This command will list all the images downloaded.
docker images

- Start the container by by below command
docker run -d -p 4444:4444 -v /dev/shm:/dev/shm selenium/standalone-chrome
Explanation of different parameters used in the above command:
docker run: docker run is the basic syntax to run the container
-d: -d is used to run the container in detached mode
-p 4444:4444: to publish a container’s port(s) to the host. First 4444 is the local machine’s port number which will be mapped with the container’s port 4444. The First 4444 can be changed depending on which port is free on your machine.
-v /dev/shm:/dev/shm: to specify volume to be bind. It shares your host machine’s memory with the container.
- To verify if the container has started successfully, run the below command. This command will return the list of all containers running.
docker ps

- Run the selenium suite. The test will execute on container only. Verify the selenium test result. To see the logs of what is happening inside the docker, use the below command. It will give you logs.
docker logs bc093155a213
In the above command, the alphanumeric string, in the end, is container id. You can get the container id from the list of the containers running. You will get logs similar to what is given in the below screenshot.

How to visualize what is happening inside container
To visualize the browser running inside a container, we can use debug images from selenium. The container running by these debug images has a VNC server running inside it on port 5900. We can connect to the same port using the VNC viewer.
Follow the below steps to run the selenium test on debug container and visualize what is the browser doing inside the container:
- Install VNC viewer on the local machine. Download the executable file from https://www.realvnc.com/en/connect/download/viewer/ and install it as per your operating system.
- Pull the debug image from docker hub selenium using the below command.
docker pull selenium/standalone-chrome-debug
- Verify the successful pull of the debug image by running the below command
docker images

- Start the container using the below command
docker run -d -p 4444:4444 -p 5901:5900 -v /dev/shm:/dev/shm selenium/standalone-chrome-debug
In above command, -p 5901:5900 is there. Below is the explanation:
-p : to declare port
5901: Local machine’s available port to map with container port on which VNC server is running. It can be any free port.
5900: Container port on which VNC server is running.
- Verify if the container is running successfully, use the below command.
docker ps

- Once the container is running, launch the VNC viewer.
- Enter address localhost:5901 in the search bar and press enter key.
- In case you see a warning about encryption click on the Continue button.
- When you are prompted to enter a password, enter ‘secret’ and click on the OK button. A new window will be launched. This is Container UI.
- Start your selenium test in IDE and observe the container view in VNC viewer just launched. You will see browser running and executing the steps you have automated.
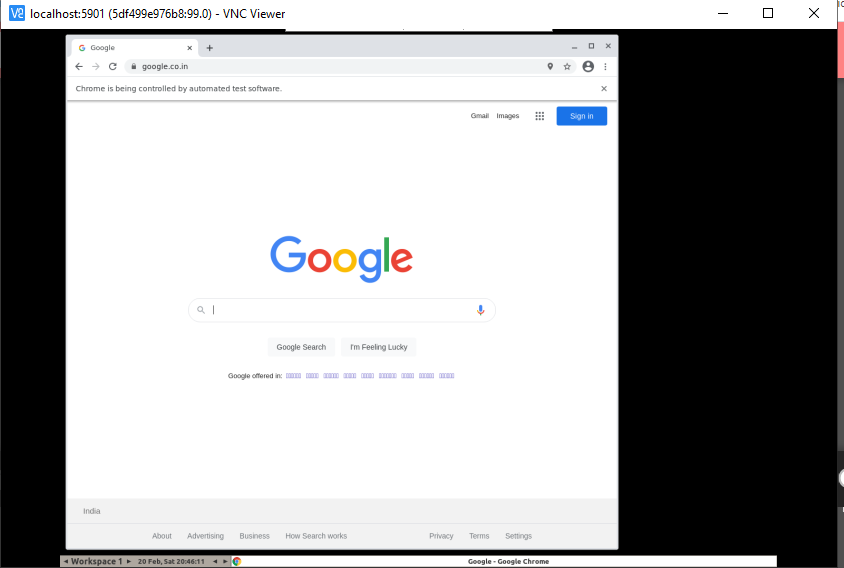
In the above steps, we have used the chrome browser for demo purposes. In the same way, we can use it for other browsers like firefox, opera, edge, etc.
We can find all the selenium images on https://hub.docker.com/u/selenium.
Conclusion
Running a selenium suite with docker is not only easy but also comes with many benefits. We can run our test on multiple environments without worrying about managing the configuration of different machines/VMs.
In this article, we learned how we can run our selenium suite on a stand-alone docker container. In the next part of the blog, we’ll see how to run a selenium grid on multiple containers with different browsers in parallel.