Leveraging Virtualization for Optimized Rendering in ReactJs
Introduction
In the realm of modern web development, achieving high performance is paramount to delivering a seamless user experience. React, a popular JavaScript library, has gained prominence for its efficient rendering capabilities. A key technique that React employs to achieve optimal rendering is virtualization.
In this article, we’ll delve into the concept of virtualization and explore its role as a powerful optimization technique for rendering in React applications.
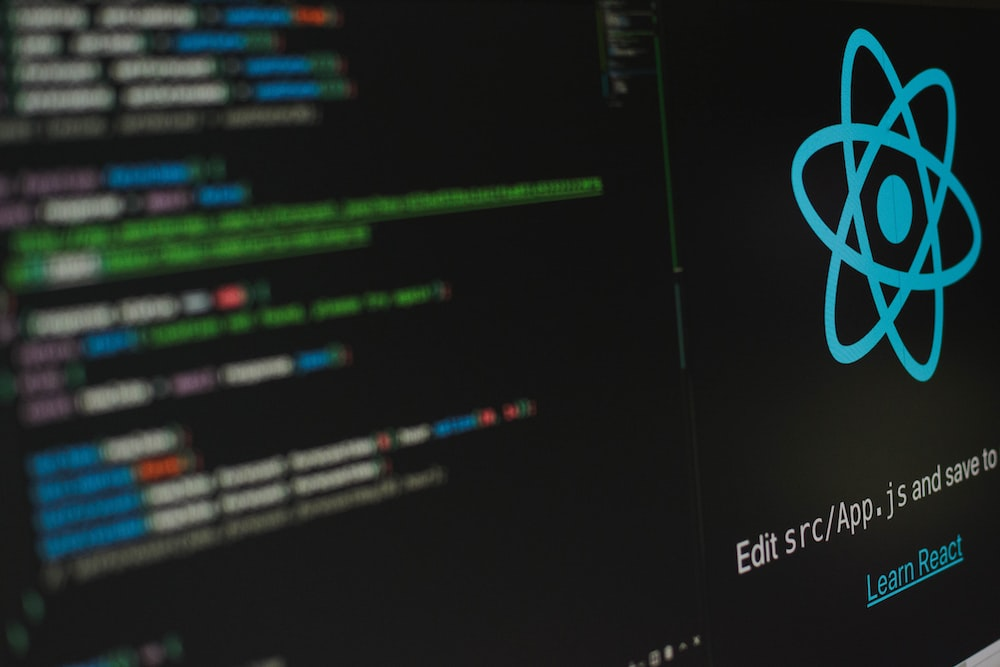
Understanding Virtualization
Definition: Virtualization is a technique used to render large datasets or collections of data by selectively rendering only the visible elements on the screen. This approach enhances performance and memory efficiency by avoiding unnecessary rendering and manipulation of off-screen components.
Benefit: Virtualization becomes particularly advantageous when dealing with lengthy lists, grids, or tables, as it ensures that the browser doesn’t become overwhelmed with excessive DOM operations.
React’s Role in Virtualization
React, with its component-based architecture and Virtual DOM, is well-suited for implementing virtualization techniques. The Virtual DOM efficiently computes and applies minimal changes required for updates, aligning perfectly with the concept of rendering only visible elements.
Two Primary Approaches to Virtualization in React:
- Windowing: Implemented using libraries like
react-window
andreact-virtualized
, this approach dynamically renders a subset of items within a scrollable container based on the user’s viewport. As the user scrolls, only the visible components are updated, significantly reducing the DOM load. - List Pagination: In this approach, large lists are divided into smaller, manageable pages. React renders only the items within the active page, achieving virtualization by limiting the number of rendered components.
Benefits of Virtualization in React
- Enhanced Performance: By rendering only visible elements, virtualization reduces initial load times and ensures smoother interactions, resulting in a more responsive user interface.
- Memory Efficiency: Traditional rendering approaches can lead to excessive memory consumption, especially with extensive datasets. Virtualization substantially reduces memory usage by rendering only the components in view.
- Improved User Experience: A responsive and fluid UI enhances user satisfaction and engagement, contributing to an overall positive experience.
- Scalability: Virtualization guarantees consistent performance as the dataset grows, making it suitable for applications with evolving and dynamic content.
- Developer-Friendly: Libraries like
react-window
abstract the complexities of virtualization, providing straightforward components to handle efficient rendering.
Implementation Examples
Example 1: Windowing with react-window
import React from 'react';
import { FixedSizeList } from 'react-window';
const LargeList = ({ data }) => {
const rowRenderer = ({ index, style }) => (
<div style={style}>{data[index]}</div>
);
return (
<FixedSizeList
height={400}
itemCount={data.length}
itemSize={50}
width={300}
>
{rowRenderer}
</FixedSizeList>
);
};
export default LargeList;
In this example, we’re using the FixedSizeList
component from react-window
to render a large list efficiently. The itemCount
and itemSize
props specify the total number of items and the height of each item, respectively. The rowRenderer
function is responsible for rendering individual items, and it’s only called for the items that are currently visible within the viewport.
Example 2: List Pagination
import React, { useState } from 'react';
const PaginatedList = ({ data, itemsPerPage }) => {
const [currentPage, setCurrentPage] = useState(1);
const startIndex = (currentPage - 1) * itemsPerPage;
const endIndex = startIndex + itemsPerPage;
const visibleItems = data.slice(startIndex, endIndex);
const handlePageChange = (newPage) => {
setCurrentPage(newPage);
};
return (
<div>
<ul>
{visibleItems.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<div>
<button
onClick={() => handlePageChange(currentPage - 1)}
disabled={currentPage === 1}
>
Previous Page
</button>
<button
onClick={() => handlePageChange(currentPage + 1)}
disabled={endIndex >= data.length}
>
Next Page
</button>
</div>
</div>
);
};
export default PaginatedList;
In this example, we’ve implemented a paginated list that renders a subset of items on each page. The data
prop contains the entire list of items, and the itemsPerPage
prop determines how many items are displayed per page. The currentPage
state variable keeps track of the currently displayed page. The handlePageChange
function allows the user to navigate between pages, ensuring that only a limited number of items are rendered at a time.
Conclusion
Virtualization stands out as a potent technique for optimizing rendering in React applications. By selectively rendering only visible components, virtualization enhances performance, memory efficiency, and user experience. React’s architectural features, combined with libraries like react-window
, enable developers to seamlessly integrate virtualization into their projects. As you strive for high-performing and responsive web applications, virtualization should be a go-to strategy in your optimization toolbox.