Email validation with Selenium
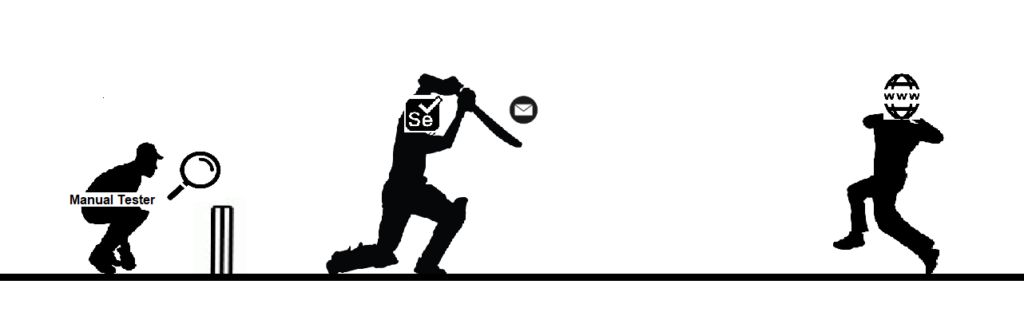
Overview
Most of the internet application take unique email ID for registration and send a verification link on the email ID to continue using the application. Also, to reset password using forgot password link, applications send a reset password link on registered email Id where user needs to click on the link to reset password. The QA teams leave such tasks for manual testing instead of writing automation tests for these scenarios. But these communication from product can be automated using disposable emails. This post will describe how to use disposable email within automation suite using selenium.
Use Cases
Here at BigShyft, to complete recruiter registration, user executes below steps:
- Enter recruiter name, company name, email ID and mobile number
- Email to verify registration is sent to email ID mentioned in step 1
- Open your email
- Click on registration confirmation link sent in the email
- Complete the registration by completing next steps
Similarly, to reset password, a reset password link is sent to user’s email ID
How we do it?
We use the disposable email service provided by mailsac.com. We can take any email id ending with ‘@mailsac.com’ e.g. test1234@mailsac.com. To check the email sent to this email ID, navigate to https://mailsac.com/inbox/test1234@mailsac.com. You will see all the emails sent to this address.
Note that all the emails sent to this address are public and anyone can use it. So use your email ID wisely (using some random string in the email ID). Also if your email contains confidential information, please avoid such mailboxes.
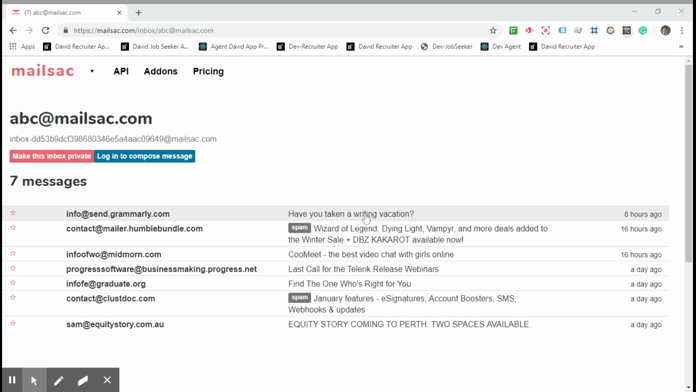
Once the email is there, you can expand the same and click on the ‘Unblock links and images’ button in the email.
It’ll open the email in the new tab where you can verify the content of the email and take action as required.
How we do it using selenium?
To send the email from our product, we execute the test automation suite which triggers the email with a registration confirmation link.
Identify the Web element on mailsac.com
Below code snippet is used to identify the elements on mailsac.com. We have used page factory approach for the same
@FindBy(xpath = "//tr[@ng-repeat = 'msg in messages']/td[4]")
public List<WebElement> list_Subjects;
//List of subjects of all emails in the inbox
@FindBy(xpath = "//button[text() = 'Delete...']")
public List<WebElement> btn_Delete;
// list of delete button on the page
@FindBy(xpath = "//button[text() = 'Permanently delete']")
public List<WebElement> btn_PermanentlyDelete;
//list of permanently delete button on the page
@FindBy(xpath = "//h3[text() = '0 messages']")
public WebElement txt_ZeroMessages;
//Text showing '0 messages' in case no message in the inbox
Create method to verify if email is received
Below code snippet can be used to verify if the email with the known subject is received in the mailbox or not.
public boolean isEmailReceived(String emailID, String subjectOfEmail) { boolean emailReceived = false; for (int j = 0; j < 10; j++) { driver.get("https://mailsac.com/inbox/" + emailID); for (int i = 0; i < list_Subjects.size(); i++) { if (list_Subjects.get(i).getText().equalsIgnoreCase(subjectOfEmail)) { emailReceived = true; break; } } if (emailReceived) { break; } else { waitHardCoded(1); } } return emailReceived; }
Create Method to delete existing emails
Below code snippet can be used to delete all existing emails in the inbox.
public void deleteAllEmail(String emailID) {
driver.get("https://mailsac.com/inbox/" + emailID);
JavascriptExecutor jse = (JavascriptExecutor) driver;
if (!isElementDisplayed(txt_ZeroMessages)) {
if (btn_Delete.size() > 0) {
for (int i = 0; i < btn_Delete.size(); i++) {
jse.executeScript("arguments[0].click();",btn_PermanentlyDelete.get(0));
waitHardCoded(1);
}
}
}
}
Open email in browser with unblocked images and links
Below code snippet can be used to open the email with all the images and links unblocked so that the user can add assertions and take action on the links given in the email.
public void openEmailInBrowser(String subject) { String dirtyURL = null; for (int i = 0; i < list_Subjects.size(); i++) { if (list_Subjects.get(i).getText().equalsIgnoreCase(subject)) { dirtyURL = driver.findElement(By.xpath("//tr[@ng-repeat = 'msg in messages']/td[text() = '" + subject + "']" + "/preceding-sibling::td//a[contains(text(), 'Unblock links and images')]")).getAttribute("href"); break; } } driver.navigate().to(dirtyURL); }
Once you open the email in the browser you can add assertions, take actions on the email as per your use case.
Conclusion
By using the disposable emails, you can verify all mail communication using selenium within the browser itself. Mailsac also provides API to test the same functionality without launching it in the browser. But there is some limit on how many API calls can be made in free account. There are few other disposable email boxes as well like mailinator.com, yopmail.com, etc.
Hi Sheelendra
Every organization is using lot of emails as medium of communication with customer for verification and promotional purposes. This has become a bigger problem in organizations to automate emails and people usually drop it for manual testing.
I was looking for a good disposal email provider and this blog completes my search.
Kudos to Sheelendra, excellent explanation with clear understanding of problem statement.
Thanks Akash….